The Myth You Should Know About && (Short Circuit Syntax) in JSX
While writing code in React, specifically in JSX, most of the junior developers (including me πΆ), have a misconception regarding && syntax. Sometimes, this misconception leads to some unavoidable bugs.
The Misconception
Have a look at the following code block.
let printHello = true;
printHello && console.log('Hello');
printHello = false;
printHello && console.log('Hello');
Can you guess the output of this code block? Most of the beginners will say the output will be β
Hello
For line 3, it will print "Hello"
and nothing for line 7. But that's not right. The output will be β
Hello
false
And thatβs the misconception, most of the beginners have regarding && syntax.
Right Concept
What actually happened in the above code block is β
-
Compiler executed the first statement
printHello
and checked whether the returned value of this statement is true or false -
When true, it executed the second statement
console.log("Hello")
-
When false, it didnβt log in to the console and returned the value of
printHello
i.e false
So && syntax will execute the 2nd argument if the first one returns true, otherwise, it will execute only the 1st one.
How JSX evaluate && syntax
JSX avoids the boolean values/undefined/null
to be rendered inside a dom element. Source That means if you write β
<div>{false}</div>
<div>{undefined}</div>
<div>{null}</div>
It will render these divs with no value.
Let think about the following React code block.
import { StrictMode } from 'react';
import ReactDOM from 'react-dom';
const rootElement = document.getElementById('root');
const isTure = true;
const isFalse = false;
const isUndefined = undefined;
const isNull = null;
function App(props) {
return <div className="App">{props.children}</div>;
}
ReactDOM.render(
<StrictMode>
<App>
<h1>Hello, I am a child</h1>
<h2>I am also a child</h2>
{isTure && <div>I will be rendered when the first statement is true</div>}
{isFalse && <h2>I will be rendered when the first statement is true</h2>}
{isUndefined && <h2>I will be rendered when the first statement is true</h2>}
{isNull && <h2>I will be rendered when the first statement is true</h2>}
</App>
</StrictMode>,
rootElement
);
It will render only the first 3 elements. Because the last 3 have false, undefined, and null as the output of the first statement. If you check out the React Dev Tools (I attached the environment at the end of this article. You can test it there), you will see false, undefined, and null as the child of App Component.
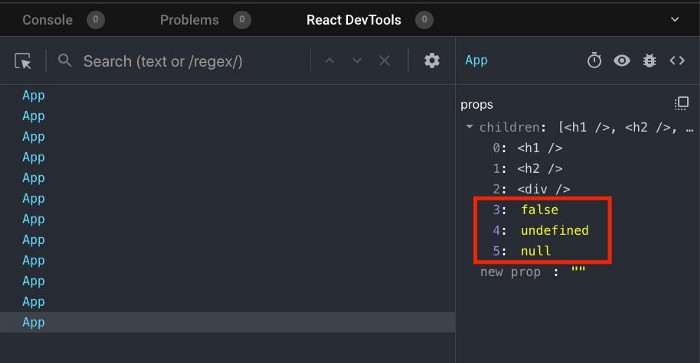
But JSX ignores these children (false, undefined, and null) while rendering.
Problem Happens Due to The Misconception
Due to the misconception regarding && syntax, most of the devs think that if we put a falsy value as the first statement, JSX will return nothing. This thinking is partially true. Because, if the falsy value is a number like 0 (while checking the length of an array is 0 or not), then JSX will avoid the second statement but not the 0. Then you will find the 0 in your browser like the following.
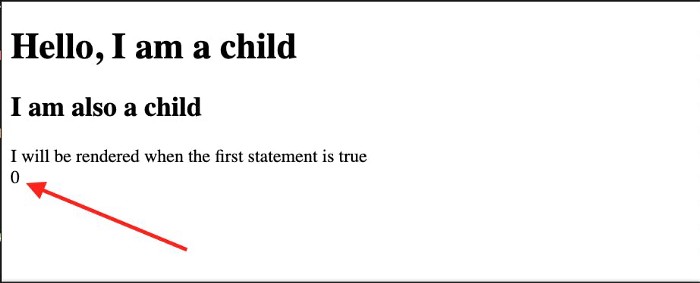
I think this is a common problem every React Dev experiences.
So, always try to put boolean values as the first statement for && syntax in JSX. Because JSX will render number even it is falsy. You can simply do it β
Instead of arr.length && <div> not empty </div>
, you have to write arr.length > 0 && <div> not empty </div>
.
Hereβs the environment of the above React code block β
Resources
-
False in JSX β https://react-cn.github.io/react/tips/false-in-jsx.html
-
Inline If with Logical && Operator β https://reactjs.org/docs/conditional-rendering.html#inline-if-with-logical--operator
-
Gist 1 β https://gist.github.com/Showrin/4caae0d642a3178cbce7fa46447eef3d
-
Gist 2 β https://gist.github.com/Showrin/db31707e53d4d118ed4ea052a24f47e5
-
Code Sandbox Repo β https://codesandbox.io/s/blue-haze-l2oyg?file=/src/index.js
Thank you for reading. If you find this article useful and like it, feel free to share it so more people can take benefit from it. You can connect with me and discuss this article on LinkedIn or my website.
Happy Coding πππ